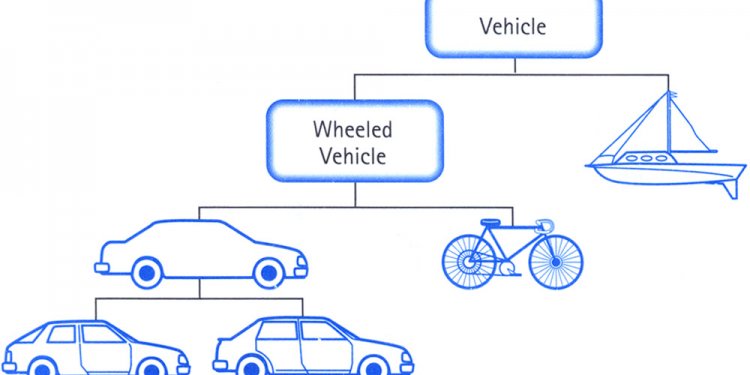
Java Object Oriented programming Interview questions
PS: If you like the page or have any questions, feel free to comment at end of the page.
Q) What are different oops concept in java?
Ans) OOPs stands for Object Oriented Programming. The concepts in oops are similar to any other programming languages. Basically, it is program agnostic.
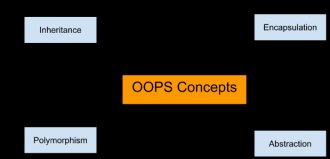
Q1) What is polymorphism?
Ans) The ability to identify a function to run is called Polymorphism. In java, c++ there are two types of polymorphism: compile time polymorphism (overloading) and runtime polymorphism (overriding).
Mehtod overriding: Overriding occurs when a class method has the same name and signature as a method in parent class. When you override methods, JVM determines the proper methods to call at the program’s run time, not at the compile time.
Overloading: Overloading is determined at the compile time. It occurs when several methods have same names with:
- Different method signature and different number or type of parameters.
- Same method signature but different number of parameters.
- Same method signature and same number of parameters but of different type
int add(int a, int b) float add(float a, int b) float add(int a, float b) void add(float a) int add(int a) void add(int a) //error conflict with the method int add(int a)
class BookDetails { String title; setBook(String title){} } class ScienceBook extends BookDetails { setBook(String title){} //overriding setBook(String title, String publisher, float price){} //overloading }
Q2) What is inheritance?
Ans) Inheritance allows a Child class to inherit properties from its parent class. In Java this is achieved by using extends keyword. Only properties with access modifier public and protected can be accessed in child class.
public class Parent { public String parentName; public String familyName; protected void printMyName { System.out.println(“ My name is “+ chidName+” “ +familyName); } } public class Child extends Parent { public String childName; public int childAge; //inheritance protected void printMyName { System.out.println(“ My child name is “+ chidName+” “ +familyName); } }
In above example the child has inherit its family name from the parent class just by inheriting the class. When child object is created printMyName present in child class is called.
Q3) What is multiple inheritance and does java support?
Ans) If a child class inherits the property from multiple classes is known as multiple inheritance. Java does not allow to extend multiple classes. The problem with with multiple inheritance is that if multiple parent classes has a same method name, the at runtime it becomes diffcult for compiler to decide which method to execute from the child class. To overcome this problem it allows to implement multiple Interfaces. The problem is commonly referred as What is Diamond Problem.